The RESTful API/REST API is the application of the API(Application Programming Interface). REST(Representational State Transfer)is an architecture of communication methods that uses the HTTP protocol for data exchange where this method is often applied in application development. With the aim to make the system have good performance, fast and easy to develop (scale) especially in the exchange and communication of data.
Building a REST API in Yii is actually quite easy. You can take advantage of the existing MVC framework, but you create different access points that you want to access by different types of services (not website visitors).
Here, we use an example to illustrate how you can build a set of RESTful APIs with little coding effort. The Yii we will use is the yii2 advanced template.
Assume you want to expose user data via RESTful APIs. The user table in this example you created when you first installed Yii. For how to install Yii you can see on How to Install Yii2 Advanced via Composer.
Steps to Create a RESTful API on Yii2 Advanced
A. Create endpoint APIs
- Copy the backend directory and rename it to “api”.
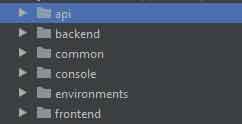
- Edit the“common/config/bootstrap.php”file and add an alias for “api.
<?php Yii::setAlias('@common', dirname(__DIR__)); Yii::setAlias('@frontend', dirname(dirname(__DIR__)) . '/frontend'); Yii::setAlias('@backend', dirname(dirname(__DIR__)) . '/backend'); Yii::setAlias('@api', dirname(dirname(__DIR__)) . '/api'); Yii::setAlias('@console', dirname(dirname(__DIR__)) . '/console');
- In the “environments/dev” directory, copy the backend directory and rename it to “api”. Likewise for the “environments / prod” directory, so it will look like the following image
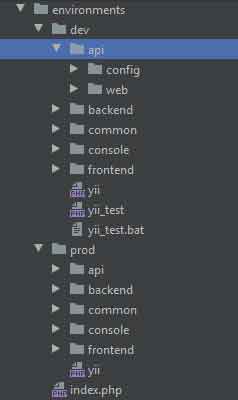
- Adjust the “environments/index.php” file as follows
return [ 'Development' = > [ 'path' = > 'dev', 'setWritable' = > [ .........., 'api/runtime', 'api/web/assets', ], .............. 'setCookieValidationKey' = > [ .........., 'api/config/main-local.php', ], ], 'Production' = > [ 'path' = > 'prod', 'setWritable' = > [ .........., 'api/runtime', 'api/web/assets', ], ............. 'setCookieValidationKey' = > [ .........., 'api/config/main-local.php', ], ], ];
- In the api directory we created earlier, change all the words “backend” to “api”
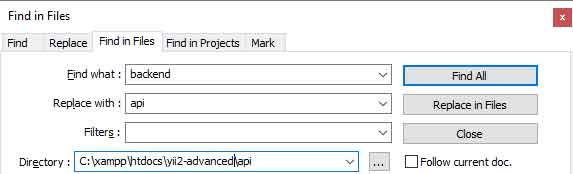
B. Yii Configuration
- Create a Controller
Create a“UserController” file in the “api/controllers” directory and copy the following scripts
<?php namespace apicontrollers; use yii\rest\ActiveController; class UserController extends ActiveController { public $modelClass = 'common\models\User'; }
- Configuration of RULES AND JSON Input URL
Change the “api/config/main.php” file by adding the JSON request and URL Rule as follows:
return [ ......... 'components' => [ 'request' => [ 'parsers' => [ 'application/json' => 'yiiwebJsonParser', ] ], ......... 'urlManager' => [ 'enablePrettyUrl' => true, 'enableStrictParsing' => true, 'showScriptName' => false, 'rules' => [ ['class' => 'yii\rest\UrlRule', 'controller' => 'user'], ], ] ............ ], ............ ];
- Create a “.htaccess” file in the “api/web/” directory and copy the following code
RewriteEngine on RewriteCond %{REQUEST_FILENAME} !-f RewriteCond %{REQUEST_FILENAME} !-d RewriteRule . index.php
C. API testing
- Download Postman,then open Postman. Make a request to the “http://localhost/yii2-advanced/api/web/users” URL
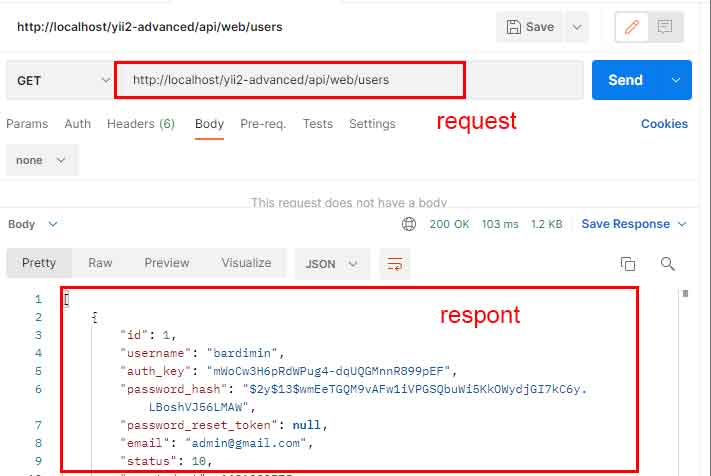
- Or with the CURL command
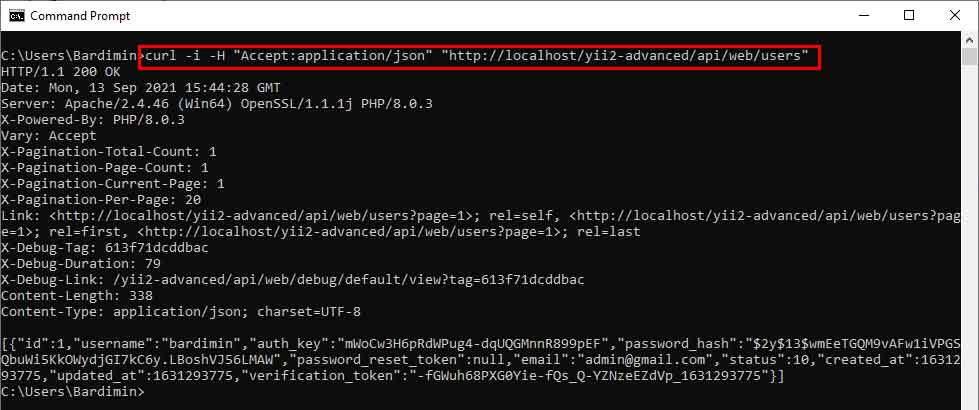
- You can also access it through a browser with the URL “”http://localhost/yii2-advanced/api/web/users”
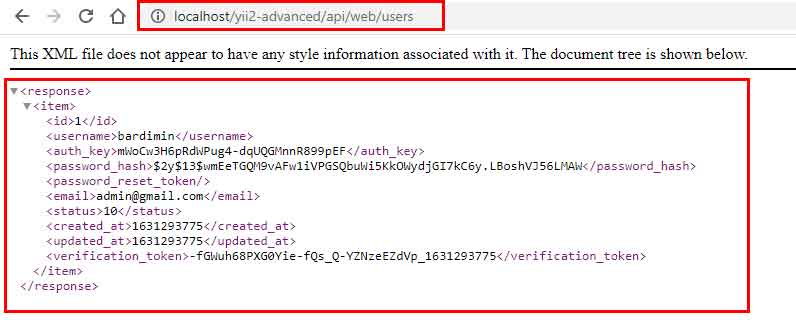