VBA (Visual Basic for Applications) is a programming language integrated into Microsoft Office applications such as Word, Excel, and PowerPoint. With VBA, users can automate manual tasks, increasing efficiency and productivity. The main advantages of using VBA include time savings, increased accuracy, and the flexibility to tailor it to specific needs.
VBA is essential for workplace productivity. Users can manage big data, create automated reports, and structure documents in a consistent format. For example, in a monthly sales report, VBA can automatically collect data, calculate total sales, and generate charts.
VBA’s daily use is very diverse. In Excel, it can be used for automated financial reports and data processing with pivot tables. In Word, users can create automated template documents and manage document elements. In PowerPoint, VBA allows you to organize presentation slides from Excel data more efficiently.
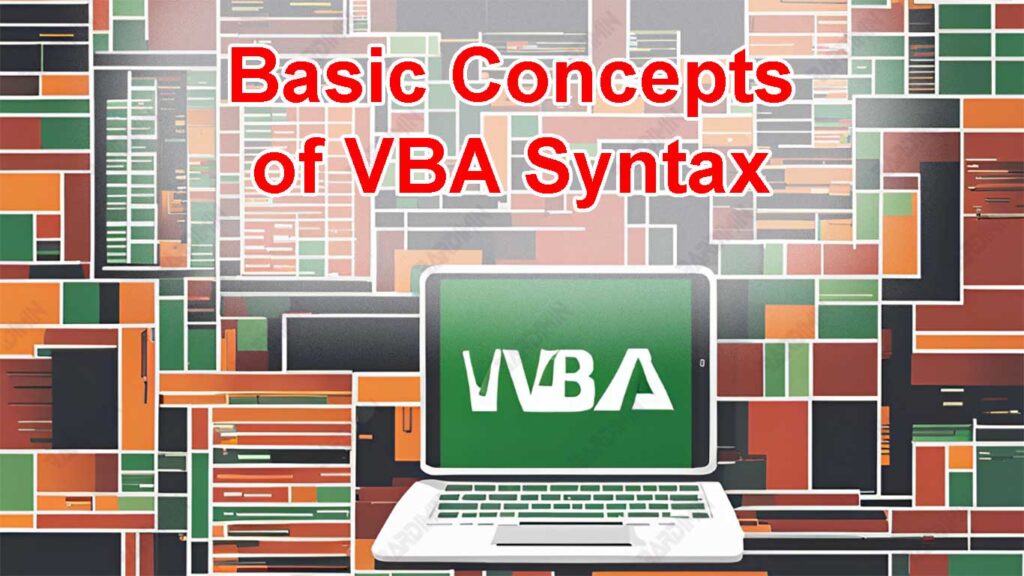
1. Understanding the Procedure in VBA
In Visual Basic for Applications (VBA), there are two main types of procedures for executing code: Subprocedures (or subs) and Functions.
a. Subprocedures
A subprocedure is a piece of code that is created to perform a specific task without returning a value. Subs are often used to execute a series of commands or macros that perform actions, such as changing the format of a document or displaying a message.
b. Functions
Functions is a procedure that also performs a specific task, but returns a value. Functions can be used in expressions and provide results that can be used again in code.
Difference between Subprocedures and Functions
Aspects | Sub | Function |
Use | Perform specific tasks | Perform tasks and return values |
Early Writing | Starting with Sub | Starting with a Function |
Return of Value | Doesn’t return a value | Returning a value with a Return |
Usage Examples | Running macros, modifying documents | Calculate the results of calculations or validate data |
Here is a simple example to understand Sub:
Sub HelloWorld()
' Displays the message “Hello, World!” in a dialog box
MsgBox “Hello, World!”
End Sub
Explanation:
- Sub HelloWorld(): Defines a procedure named “HelloWorld”.
- MsgBox: VBA built-in commands to display a dialog box containing messages.
- End Sub: Marks the end of the Sub procedure.
For Function, here’s a simple example:
Function AddNumbers(ByVal a As Integer, ByVal b As Integer) As Integer
' Returns the sum of two numbers
AddNumbers = a + b
End Function
This function can be called in other code or directly in Excel, such as using a regular formula:
=AddNumbers(5, 10)
2. Variables and Constants
What are Variables and How to Declare Them
The variable is a place to store data that can change as the program runs. In VBA, a variable must be declared before it can be used with the Dim keyword, followed by the name of the variable and its data type. For example, to declare a variable of type string, you can use the following syntax:
Dim name As String ' Stores text
Dim age As Integer ' Stores integer
Dim height As Double ' Stores decimal number
By declaring variables, you tell VBA about the type of data to be stored, which helps in memory management and makes the code clearer.
The Importance of Using Option Explicit
Using Option Explicit at the beginning of a VBA module is highly recommended. By adding this statement, you are required to declare all variables before using them. This helps avoid typos and logical errors that often arise due to the use of undeclared or misspelled variables. Examples of use:
Option Explicit
Sub Example()
Dim age As Integer
age = 25
End Sub
With Option Explicit, if you try to use a variable without declaring it first, VBA will give an error, thus helping to maintain the quality of the code.