Errors Handling is a vital aspect of Excel VBA programming, especially when users enter invalid values on a worksheet. Here is a guide on how to handle frequently occurring errors in Excel VBA.
Errors handling not only prevents the program from crashing due to incorrect input, but also:
- Provide clear feedback to users regarding errors that occur
- Ease code maintenance and troubleshooting
By handling errors well, programmers can ensure that the program runs smoothly and that users have a better experience.
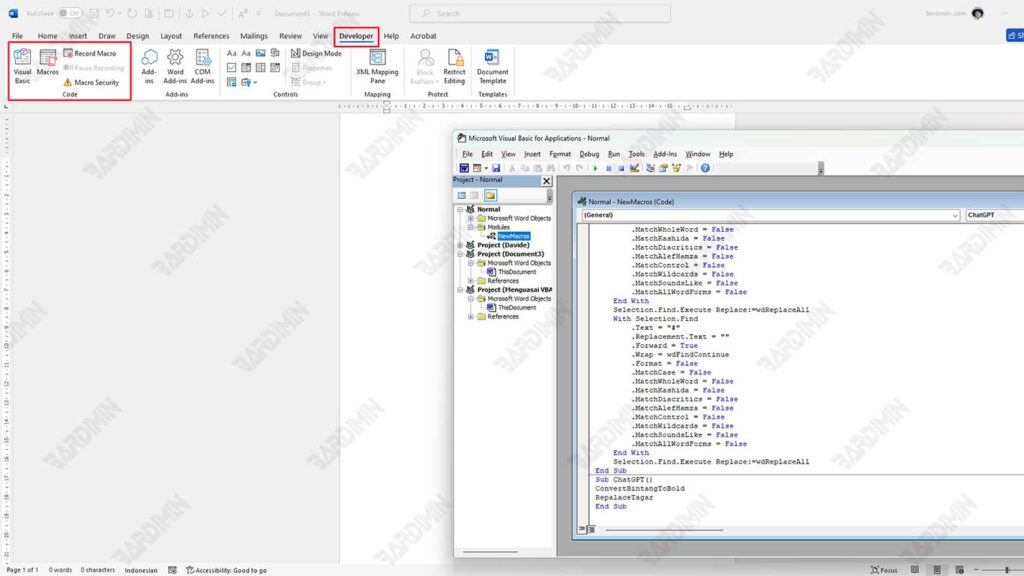
In the world of Excel VBA programming, errors can appear anytime a user enters an invalid value into a worksheet. These errors, if not handled properly, can cause the program to stop or give unwanted results.
In this article, Bardimin will discuss various methods to identify and fix common errors in Excel VBA, as well as offer practical solutions to keep the program running smoothly despite interruptions.
Types of Errors and How to Write Errors Handling Code
Error handling in Excel VBA is essential to ensure the program runs properly and provides a positive user experience. Here are some common types of error handling used in Excel VBA:
1. On Error GoTo
This type of error handling is used to direct a program to a specific piece of code when an error occurs. Once detected, the program flow will move to a specific label that has been defined to handle the error.
Private Sub CommandButton1_Click()
On Error GoTo err_handler
num1 = InputBox("Enter the first number")
num2 = InputBox("Enter the second number")
MsgBox num1 / num2
Exit Sub
err_handler:
MsgBox "Invalid division, please try again"
End Sub
In this example, if an error occurs (for example, division by zero), the program will jump to the err_handler label and display an error message.
2. On Error Resume Next
With On Error Resume Next, the program will ignore the error and continue executing the code on the next line. This is useful for non-critical errors that don’t require special handling.
Private Sub CommandButton2_Click()
On Error Resume Next
num1 = InputBox("Enter the first number")
num2 = InputBox("Enter the second number")
MsgBox num1 / num2
End Sub
Here, even if there is a division by zero, the program will not stop, and the execution will continue to the next line.
The use of Resume Next should be done with caution, as it can hide important errors.
3. On Error GoTo 0
On Error GoTo 0 is used to disable error handling that was previously enabled. After using this command, if there is an error on the next line, the program will stop and display a standard error message.
Sub ExampleGoToZero()
On Error Resume Next ' Ignore errors
Dim result As Double
result = 5 / 0 ' Errors are ignored
On Error GoTo 0 ' Disable error handling
MsgBox result
result = 5 / 0 ' This will cause the program to terminate since errors are no longer ignored
End Sub
After using On Error GoTo 0, if an error occurs, the program will stop as usual.
4. On Error GoTo -1
This type is used to reset the error handling status that applies in the scope of the current procedure. Errors that were previously handled will no longer affect the program flow.
Sub ExampleGoToZero()
On Error Resume Next ' Ignore errors
Dim result As Double
result = 5 / 0 ' Errors are ignored
On Error GoTo 0 ' Disable error handling
MsgBox result
result = 5 / 0 ' This will cause the program to terminate since errors are no longer ignored
End Sub
With On Error GoTo -1, the previous error handling will be removed, so you can set up new handling if necessary.