Merging cells in Excel is useful when you want to combine multiple cells into one larger cell. It can be used to title, organize data, or format the look of your worksheet.
Excel VBA is a programming language used to automate tasks in Microsoft Excel. One task that is often done in Excel is to combine cells, to unite several cells into one large cell. Merging cells can be useful for creating specific headings, tables, or data formats.
There are several ways to combine cells using Excel VBA, namely:
- Use the “Merge” property of the “Range” object.
- Use the “MergeCells” method of the “Range” object.
- Use the “MergeArea” method of the “Range” object.
- Use the “UnMerge” method of the “Range” object to unmerge cells.
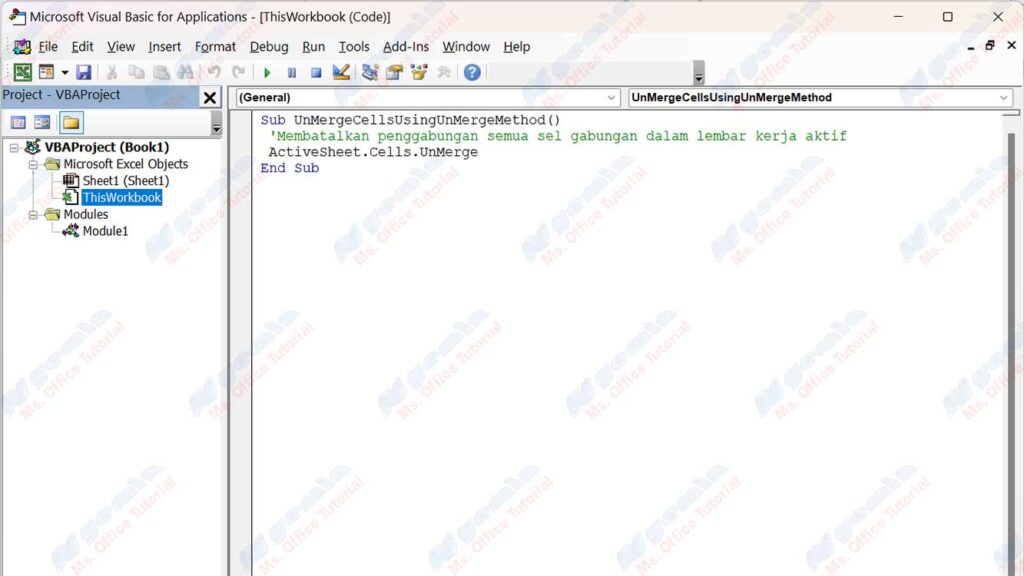
This article will cover these methods in detail, along with code examples and results. We will also discuss some things to note when merging cells using Excel VBA.
Using the “Merge” Property of a “Range” Object
The “Merge” property of the “Range” object is used to set or return a Boolean value, indicating whether a range of cells is combined or not. A value of “True” means a range of cells is merged, while a False value means a range of cells is not merged.
To merge cells using the “Merge” property, we can assign “True” values to those properties for the range of cells we want to combine. The following code sample shows how to merge cells A1 through C3 using the Merge property.
Sub MergeCellsUsingMergeProperty() 'Combining cells A1 to C3 Range( “A1:C3” ). Merge = True End Sub
We can see that cells A1 through C3 have been merged into one large cell, and the value from cell A1 remains displayed in the middle of the merged cell.
To unmerge cells usthe ing “Merge” property, we can assign a “False” value to that property for the range of cells we want to split. The following code sample shows how to unmerge cells A1 through C3 using the “Merge” property.
Sub UnMergeCellsUsingMergeProperty() 'Unmerge cells A1 through C3 Range( “A1:C3” ). Merge = False End Sub
We can see that cells A1 through C3 have been separated into individual cells, and the value from cell A1 remains displayed in cell A1.
Using the “MergeCells” Method of the “Range” Object
The “MergeCells” method of “Range” objects is used to merge or unmerge selected cell ranges. This method has no arguments and only works on the range of cells selected by the user.
To merge cells using the “MergeCells” method, we can select the range of cells we want to combine, and then run the method. The following code sample shows how to combine cells D1 through F3 using the “MergeCells” method.
Sub MergeCellsUsingMergeCellsMethod() 'Select cells D1 through F3 Range( “D1:F3” ). Select 'Merge selected cells Selection.MergeCells End Sub
We can see that cells D1 through F3 have been merged into one large cell, and the value from cell D1 remains displayed in the middle of the merged cell.
To unmerge cells using the “MergeCells” method, we can select the range of cells that we want to split, and then run the method. The following code sample shows how to unmerge cells D1 through F3 using the “MergeCells” method.
Sub UnMergeCellsUsingMergeCellsMethod() 'Select cells D1 through F3 Range( “D1:F3” ). Select 'Unmerge selected cells Selection.MergeCells End Sub
We can see that cells D1 through F3 have been separated into individual cells, and the value from cell D1 remains displayed in cell D1.