A variable is a named place in a computer’s memory that is used to store data temporarily while a program is running. There are different types of variables, such as String for text, Integer for round numbers, Boolean for true or false values, and Object for objects. Variables provide flexibility for programmers to store and process information.
A constant is a named item that stores a fixed value that does not change during the running of the program. Although many programmers use variables more often, constants are also important for storing values that should not be changed.
Enumeration is a collection of interrelated constants, allowing programmers to use names rather than numbers to refer to specific items. For example, it’s easier to use a name like AnimationFlyIntoFromLeft than a number like 1312. Enumeration helps organize the code and improve readability.
Understanding the use of variables is essential in programming because variables are the basis for data storage and processing. By using variables effectively, programmers can write code that is cleaner and easier to understand.
Additionally, a good understanding of how to declare and use variables, constants, and enumerations can help avoid common mistakes and improve program efficiency. Well-structured code is not only easier to read but also easier to manage and improve in the future.
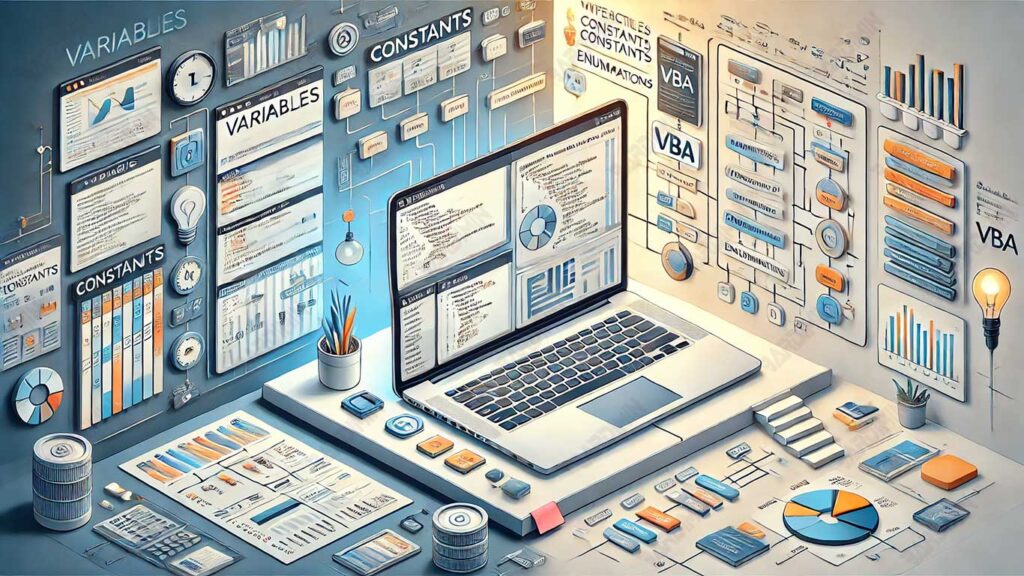
Understanding Variables
A. Types of Variables
In programming, several types of variables are frequently used, each with different characteristics and functions. Here is an explanation of these types of variables:
- String: This variable type is used to store text.
- Integer: This type is used to store round numbers.
- Boolean: This type stores true or false values.
- Date: This type is used to store date and time information.
- Object: This type is used to store objects in programming, such as forms or controls.
B. Proper Variable Naming
Correct variable naming is essential to keep the code easy to read and maintain. Here are some rules and best practices in variable naming:
1. Variable Naming Rules:
Variable names should start with a letter and can be up to 255 characters long, but it’s better to make them shorter to make them easier to type.
Variable names must not contain special characters such as periods, exclamation marks, or mathematical operators (+, -, /, *), and must not contain spaces. However, you can use an underscore (_) to separate words.
Avoid using the same name as VBA keywords or built-in functions to avoid confusion.
2. The Importance of Using Naming Conventions:
Using naming conventions like strUserName for string variables helps other programmers understand the data type just by looking at its name.
Example of a good variable name:
- userAge (to store age)
- isUserLoggedIn (to store the user’s login state)
- totalPrice (to store the total price)
C. Variable Declaration
In VBA programming, variables can be declared implicit or explicit.
Implicit Declaration
In this method, variables are used without prior declaration. VBA automatically creates a variable with that name and sets it as a Variant data type, which can store a variety of data types. Example:
myVariable = “Sample variable text”
This method’s advantage is faster code writing, but it also has drawbacks, such as undetected typing errors, which can lead to the creation of new unwanted variables.
Explicit Declaration
This method requires a variable declaration before its use with keywords such as Dim, Private, or Public. This allows programmers to specify variable data types and provide more clarity to the code. Example:
Dim myVariable As String
myVariable = “Sample variable text”
The advantages of this method include improved code readability, ease of debugging, and avoidance of typing errors.